Difference Between CompletableFuture And Future In Java
Future was introduced in Java 5 and has very limited functionality.
CompletableFuture was introduced in Java 8 with additional features like option to chain function calls onto the result of the initial task.
1. Blocking vs Non-Blocking
Future: When using
Future
, you submit a task to anExecutorService
, and it returns aFuture
object. However, to get the result of the computation, you must call theget()
method, which blocks the calling thread until the task is complete. This can make your application less responsive, as it waits for the computation to finish.CompletableFuture: With
CompletableFuture
, you get non-blocking execution. It allows you to define what happens when the computation is done through methods likethenApply()
,thenAccept()
, andthenRun()
. This makes your application much more responsive, as it doesn't block the main thread while waiting for the result.
2. Callback Support
Future: Unfortunately,
Future
doesn't allow you to attach callbacks that define what to do when the computation is done. You must block the thread withget()
and manually process the result later.CompletableFuture: On the other hand,
CompletableFuture
provides full support for chaining tasks. You can attach various callbacks usingthenApply()
,thenAccept()
, orthenRun()
to define what should happen after the task completes, without blocking the main thread.
3. Exception Handling
Future: If the task fails,
Future
provides no built-in mechanism to handle exceptions. You must catch exceptions when you callget()
, making error handling a bit clunky.CompletableFuture: Offers much more robust exception handling with methods like
exceptionally()
andhandle()
. These methods allow you to define fallback actions or recovery mechanisms within the same async computation chain, ensuring smoother error management.
4. Composing Results
Future: One of the major limitations of
Future
is the inability to combine multipleFuture
instances or chain tasks. If you need to wait for multiple tasks to complete, you have to manage it manually, which can be cumbersome.CompletableFuture: This is where
CompletableFuture
excels. It allows you to compose and combine asynchronous tasks easily using methods likethenCompose()
,thenCombine()
, or run multiple tasks in parallel withallOf()
. This makes complex workflows far easier to handle.
5. Asynchronous Execution Support
Future: To run tasks asynchronously,
Future
needs to be paired with anExecutorService
, which adds some overhead. While this approach works for simple scenarios, it lacks the flexibility of more advanced async handling.CompletableFuture: With
CompletableFuture
, asynchronous execution is built-in. Methods likesupplyAsync()
andrunAsync()
allow you to run tasks asynchronously without needing to explicitly manage anExecutorService
.
6. Use Cases
Future: Use
Future
for simple use cases where you need to execute a task in another thread and block until you retrieve the result. It's helpful for basic concurrency but lacks advanced features.CompletableFuture: If you need to handle more complex asynchronous workflows with non-blocking behavior, error handling, and task composition,
CompletableFuture
is the way to go. It offers a more complete solution for modern async programming in Java.
Below is the summary of methods available in Completable Future:
CompletableFuture
.CompletableFuture
once it completes.CompletableFuture
after completion without returning a value.CompletableFuture
completes without using its result.CompletableFuture
tasks sequentially by using the result of the first to trigger the second.CompletableFuture
tasks and processes them together.CompletableFuture
tasks to complete and combines them.CompletableFuture
task to complete, then proceeds.CompletableFuture
.CompletableFuture
with a result.CompletableFuture
exceptionally with a given error.get()
, but throws unchecked exceptions, making it more convenient to use in async chains.CompletableFuture
and triggers an exception if exceeded.CompletableFuture
with a default value if the task takes too long.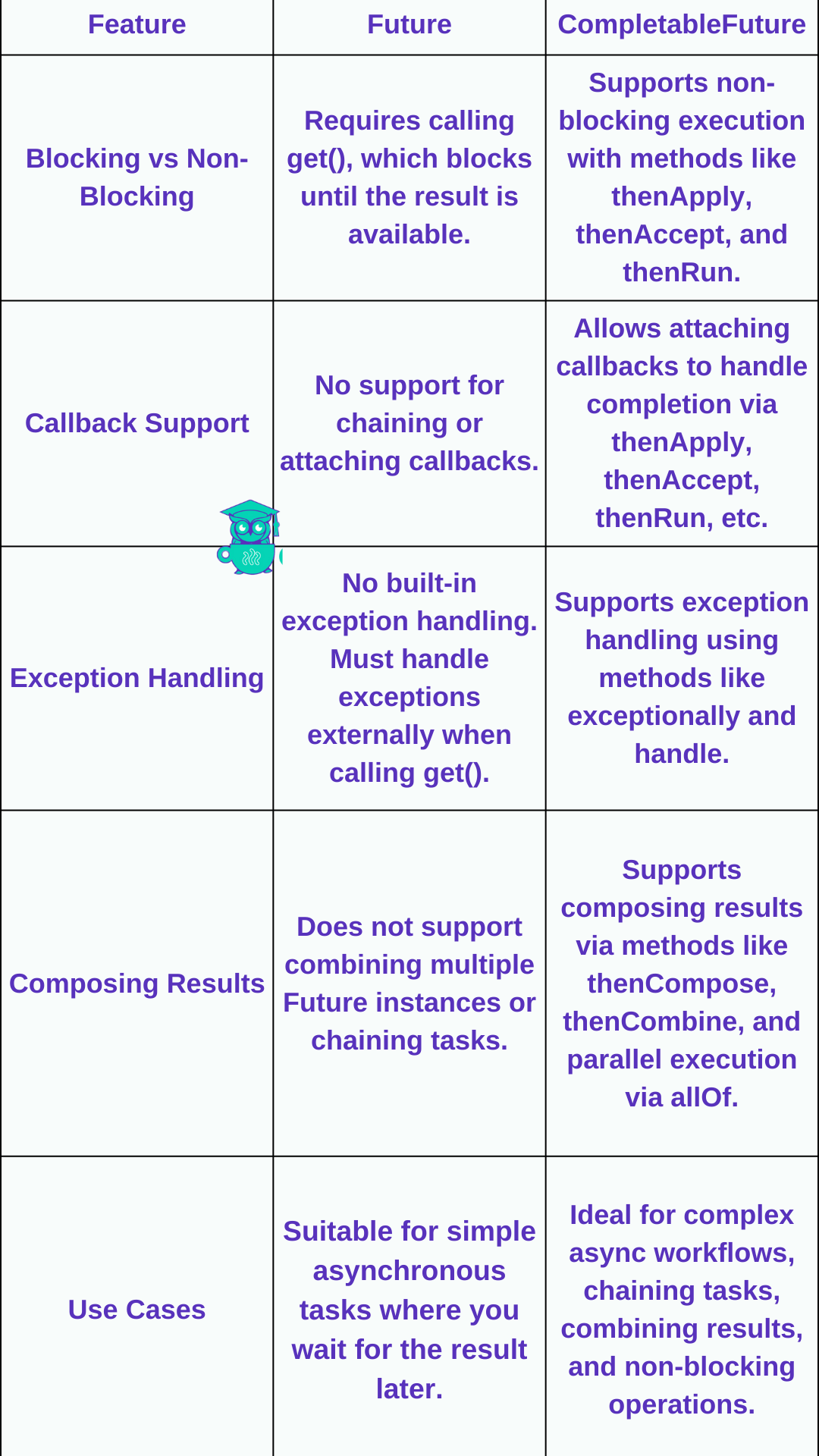
Who we are
Courses
-
Java Spring Boot Course
-
System Design Course
-
Blogs
-
Join Our Team
-
Newsletter